Understanding the Event Loop in Node.js
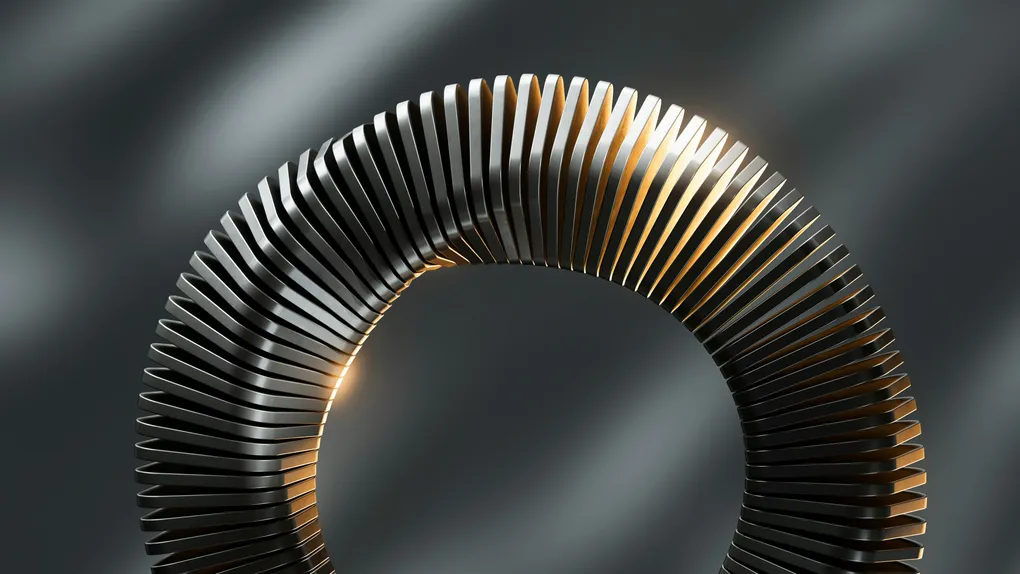
When it comes to understanding the core of Node.js’s asynchronous and non-blocking I/O operations, the Event Loop is a fundamental concept to grasp. It’s what enables Node.js to handle high concurrency without the need for multiple threads, making it an excellent choice for building scalable and performant applications.
What is the Event Loop?
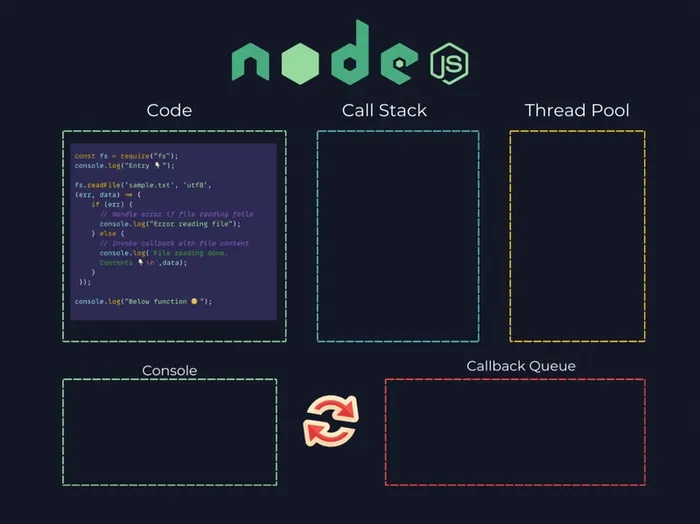
At its core, the Event Loop is a mechanism responsible for handling asynchronous operations in Node.js. It allows Node.js to perform I/O operations without blocking the execution of other tasks. The Event Loop continuously checks if there are any tasks in the queue, and if so, it executes them one by one.
How Does the Event Loop Work?
Understanding the Event Loop involves grasping the concept of phases and the order of operations within each phase:
-
Timers: This phase executes callbacks scheduled by
setTimeout()
andsetInterval()
. -
Pending Callbacks: In this phase, I/O callbacks deferred to the next loop iteration are executed.
-
Idle, Prepare: These are internal phases used by the Event Loop.
-
Poll: This phase retrieves new I/O events; executes I/O-related callbacks such as networking requests, file system operations, etc. If the Event Loop has no work to do, it may block and wait for events.
-
Check: This phase executes
setImmediate()
callbacks. -
Close Callbacks: This phase executes close event callbacks, such as
socket.on('close', ...)
.
The Event Loop continuously iterates over these phases, handling various types of asynchronous operations.
Event Loop in Action
Let’s visualize how the Event Loop works with a simple example:
console.log("Start");
setTimeout(() => {
console.log("Timer callback");
}, 0);
setImmediate(() => {
console.log("Immediate callback");
});
console.log("End");
When you run this code, the output might be surprising:
Start
End
Immediate callback
Timer callback
Despite setTimeout()
being called before setImmediate()
, the Immediate callback is executed first. This is because setImmediate()
queues its callback in a separate phase of the Event Loop, making it execute before the callback from setTimeout()
.
Conclusion
Understanding the Event Loop is crucial for writing efficient Node.js applications. It enables developers to take full advantage of Node.js’s asynchronous and non-blocking nature, allowing for high concurrency and better scalability. By mastering how the Event Loop operates, developers can build performant applications that handle I/O operations seamlessly, making Node.js a powerful platform for various use cases. *generated by chatGPT